- All Implemented Interfaces:
Shape
,Serializable
,Cloneable
,Emptiable
,Envelope
This class inherits x and y fields. But despite their names, they don't need to be oriented toward East and North respectively. The (x,y) axis can have any direction and should be understood as coordinate 0 and coordinate 1 values instead. This is not specific to this implementation; in Java2D too, the visual axis orientation depend on the affine transform in the graphics context.
Crossing the anti-meridian of a Geographic CRS
The Web Coverage Service (WCS) specification authorizes (with special treatment) cases where upper < lower at least in the longitude case. They are envelopes crossing the anti-meridian, like the red box below (the green box is the usual case). ForEnvelope2D
objects, they are rectangle with negative width or
height field values. The default implementation of methods listed in the
right column can handle such cases.
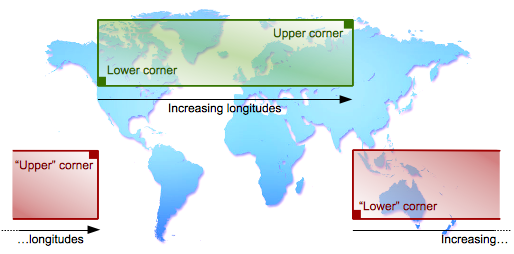
getMinimum(int)
getMaximum(int)
getSpan(int)
getMedian(int)
isEmpty()
toRectangles()
contains(double,double)
contains(Rectangle2D)
and its variant receivingdouble
argumentsintersects(Rectangle2D)
and its variant receivingdouble
argumentscreateIntersection(Rectangle2D)
createUnion(Rectangle2D)
add(Rectangle2D)
add(double,double)
getMinX()
, getMinY()
, getMaxX()
, getMaxY()
,
getCenterX()
, getCenterY()
, getWidth()
and getHeight()
methods delegate to the above-cited methods.- Since:
- 0.3
- See Also:
-
Nested Class Summary
Nested classes/interfaces inherited from class Rectangle2D
Rectangle2D.Double, Rectangle2D.Float
-
Field Summary
Fields inherited from class Rectangle2D.Double
height, width, x, y
Fields inherited from class Rectangle2D
OUT_BOTTOM, OUT_LEFT, OUT_RIGHT, OUT_TOP
-
Constructor Summary
ConstructorsConstructorDescriptionConstructs an initially empty envelope with no CRS.Envelope2D
(DirectPosition lowerCorner, DirectPosition upperCorner) Constructs a two-dimensional envelope defined by the specified coordinates.Envelope2D
(Envelope envelope) Constructs a two-dimensional envelope defined by anotherEnvelope
.Constructs a new envelope with the same data than the specified geographic bounding box.Envelope2D
(CoordinateReferenceSystem crs, double x, double y, double width, double height) Constructs two-dimensional envelope defined by the specified coordinates.Envelope2D
(CoordinateReferenceSystem crs, Rectangle2D rect) Constructs two-dimensional envelope defined by anotherRectangle2D
. -
Method Summary
Modifier and TypeMethodDescriptionvoid
add
(double px, double py) Adds a point to this rectangle.void
add
(Rectangle2D rect) Adds another rectangle to this rectangle.boolean
boundsEquals
(Envelope that, int xDim, int yDim, double eps) Returnstrue
ifthis
envelope bounds is equal tothat
envelope bounds in two specified dimensions.clone()
Returns a clone of this envelope.boolean
contains
(double px, double py) Tests if a specified coordinate is inside the boundary of this envelope.boolean
contains
(double rx, double ry, double rw, double rh) Returnstrue
if this envelope completely encloses the specified rectangle.boolean
contains
(Rectangle2D rect) Returnstrue
if this envelope completely encloses the specified rectangle.Returns the intersection of this envelope with the specified rectangle.createUnion
(Rectangle2D rect) Returns the union of this envelope with the specified rectangle.boolean
Compares the specified object with this envelope for equality.double
Returns the median coordinate value for dimension 0.double
Returns the median coordinate value for dimension 1.Returns the coordinate reference system in which the coordinates are given.final int
Returns the number of dimensions, which is always 2.double
Returns the span for dimension 1.The limits in the direction of decreasing coordinate values for the two dimensions.double
getMaximum
(int dimension) Returns the maximal coordinate along the specified dimension.double
Returns the maximal coordinate value for dimension 0.double
Returns the maximal coordinate value for dimension 1.A coordinate position consisting of all the median coordinate values.double
getMedian
(int dimension) Returns the median coordinate along the specified dimension.double
getMinimum
(int dimension) Returns the minimal coordinate along the specified dimension.double
Returns the minimal coordinate value for dimension 0.double
Returns the minimal coordinate value for dimension 1.double
getSpan
(int dimension) Returns the envelope span along the specified dimension.The limits in the direction of increasing coordinate values for the two dimensions.double
Returns the span for dimension 0.boolean
intersects
(double rx, double ry, double rw, double rh) Returnstrue
if this envelope intersects the specified envelope.boolean
intersects
(Rectangle2D rect) Returnstrue
if this envelope intersects the specified envelope.boolean
isEmpty()
Determines whether the envelope is empty.void
Sets the coordinate reference system in which the coordinate are given.void
setRect
(Rectangle2D rect) Sets this envelope to the given rectangle.Returns this envelope as non-empty Java2D rectangle objects.Formats this envelope as a "BOX
" element.Methods inherited from class Rectangle2D.Double
getBounds2D, getX, getY, outcode, setRect
Methods inherited from class Rectangle2D
add, getPathIterator, getPathIterator, hashCode, intersect, intersectsLine, intersectsLine, outcode, setFrame, union
Methods inherited from class RectangularShape
contains, getBounds, getFrame, setFrame, setFrame, setFrameFromCenter, setFrameFromCenter, setFrameFromDiagonal, setFrameFromDiagonal
-
Constructor Details
-
Envelope2D
public Envelope2D()Constructs an initially empty envelope with no CRS. -
Envelope2D
public Envelope2D(DirectPosition lowerCorner, DirectPosition upperCorner) throws MismatchedReferenceSystemException, MismatchedDimensionException Constructs a two-dimensional envelope defined by the specified coordinates. ThelowerCorner
andupperCorner
arguments are not necessarily the minimal and maximal values respectively. See the class javadoc about crossing the anti-meridian for more details.- Parameters:
lowerCorner
- the first position.upperCorner
- the second position.- Throws:
MismatchedReferenceSystemException
- if the two positions don't use the same CRS.MismatchedDimensionException
- if the two positions are not two-dimensional.
-
Envelope2D
Constructs a two-dimensional envelope defined by anotherEnvelope
.- Parameters:
envelope
- the envelope to copy (cannot benull
).- Throws:
MismatchedDimensionException
- if the given envelope is not two-dimensional.
-
Envelope2D
Constructs a new envelope with the same data than the specified geographic bounding box. The coordinate reference system is set to the default geographic CRS. Axis order is (longitude, latitude).- Parameters:
box
- The bounding box to copy (cannot benull
).
-
Envelope2D
public Envelope2D(CoordinateReferenceSystem crs, Rectangle2D rect) throws MismatchedDimensionException Constructs two-dimensional envelope defined by anotherRectangle2D
. If the given rectangle has negative width or height, they will be interpreted as an envelope crossing the anti-meridian.- Parameters:
crs
- the coordinate reference system, ornull
.rect
- the rectangle to copy (cannot benull
).- Throws:
MismatchedDimensionException
- if the given CRS is not two-dimensional.
-
Envelope2D
public Envelope2D(CoordinateReferenceSystem crs, double x, double y, double width, double height) throws MismatchedDimensionException Constructs two-dimensional envelope defined by the specified coordinates. Despite their name, the (x,y) coordinates don't need to be oriented toward (East, North). Those parameter names simply match the x and y fields. The actual axis orientations are determined by the specified CRS. See the class javadoc for details.- Parameters:
crs
- the coordinate reference system, ornull
.x
- the x minimal value.y
- the y minimal value.width
- the envelope width. May be negative for envelope crossing the anti-meridian.height
- the envelope height. May be negative for envelope crossing the anti-meridian.- Throws:
MismatchedDimensionException
- if the given CRS is not two-dimensional.
-
-
Method Details
-
getCoordinateReferenceSystem
Returns the coordinate reference system in which the coordinates are given.- Specified by:
getCoordinateReferenceSystem
in interfaceEnvelope
- Returns:
- the coordinate reference system, or
null
.
-
setCoordinateReferenceSystem
Sets the coordinate reference system in which the coordinate are given. This method does not reproject the envelope. If the envelope coordinates need to be transformed to the new CRS, consider usingEnvelopes.transform(Envelope, CoordinateReferenceSystem)
instead.- Parameters:
crs
- the new coordinate reference system, ornull
.
-
setRect
Sets this envelope to the given rectangle. If the given rectangle is also an instance ofEnvelope
(typically as anotherEnvelope2D
) and has a non-null Coordinate Reference System (CRS), then the CRS of this envelope will be set to the CRS of the given envelope.- Overrides:
setRect
in classRectangle2D.Double
- Parameters:
rect
- the rectangle to copy coordinates from.- Since:
- 0.8
-
getDimension
public final int getDimension()Returns the number of dimensions, which is always 2.- Specified by:
getDimension
in interfaceEnvelope
- Returns:
- always 2 for bi-dimensional objects.
-
getLowerCorner
The limits in the direction of decreasing coordinate values for the two dimensions. This is typically a coordinate position consisting of the minimal coordinates for the two dimensions for all points within theEnvelope
.The object returned by this method is a copy. Change in the returned position will not affect this envelope, and conversely.
Note on wraparound
The Web Coverage Service (WCS) 1.1 specification uses an extended interpretation of the bounding box definition. In a WCS 1.1 data structure, the lower corner defines the edges region in the directions of decreasing coordinate values in the envelope CRS. This is usually the algebraic minimum coordinates, but not always. For example, an envelope crossing the anti-meridian could have a lower corner longitude greater than the upper corner longitude. Such extended interpretation applies mostly to axes havingWRAPAROUND
range meaning.- Specified by:
getLowerCorner
in interfaceEnvelope
- Returns:
- a copy of the lower corner, typically (but not necessarily) containing minimal coordinate values.
- See Also:
-
getUpperCorner
The limits in the direction of increasing coordinate values for the two dimensions. This is typically a coordinate position consisting of the maximal coordinates for the two dimensions for all points within theEnvelope
.The object returned by this method is a copy. Change in the returned position will not affect this envelope, and conversely.
Note on wraparound
The Web Coverage Service (WCS) 1.1 specification uses an extended interpretation of the bounding box definition. In a WCS 1.1 data structure, the upper corner defines the edges region in the directions of increasing coordinate values in the envelope CRS. This is usually the algebraic maximum coordinates, but not always. For example, an envelope crossing the anti-meridian could have an upper corner longitude less than the lower corner longitude. Such extended interpretation applies mostly to axes havingWRAPAROUND
range meaning.- Specified by:
getUpperCorner
in interfaceEnvelope
- Returns:
- a copy of the upper corner, typically (but not necessarily) containing maximal coordinate values.
- See Also:
-
getMedian
A coordinate position consisting of all the median coordinate values.The object returned by this method is a copy. Change in the returned position will not affect this envelope, and conversely.
- Returns:
- a copy of the median coordinates.
- Since:
- 1.1
- See Also:
-
getMinimum
Returns the minimal coordinate along the specified dimension. This method handles anti-meridian as documented in theAbstractEnvelope.getMinimum(int)
method.- Specified by:
getMinimum
in interfaceEnvelope
- Parameters:
dimension
- the dimension to query.- Returns:
- the minimal coordinate value along the given dimension.
- Throws:
IndexOutOfBoundsException
- if the given index is out of bounds.
-
getMaximum
Returns the maximal coordinate along the specified dimension. This method handles anti-meridian as documented in theAbstractEnvelope.getMaximum(int)
method.- Specified by:
getMaximum
in interfaceEnvelope
- Parameters:
dimension
- the dimension to query.- Returns:
- the maximal coordinate value along the given dimension.
- Throws:
IndexOutOfBoundsException
- if the given index is out of bounds.
-
getMedian
Returns the median coordinate along the specified dimension. This method handles anti-meridian as documented in theAbstractEnvelope.getMedian(int)
method.- Specified by:
getMedian
in interfaceEnvelope
- Parameters:
dimension
- the dimension to query.- Returns:
- the mid coordinate value along the given dimension.
- Throws:
IndexOutOfBoundsException
- if the given index is out of bounds.- See Also:
-
getSpan
Returns the envelope span along the specified dimension. This method handles anti-meridian as documented in theAbstractEnvelope.getSpan(int)
method.- Specified by:
getSpan
in interfaceEnvelope
- Parameters:
dimension
- the dimension to query.- Returns:
- the rectangle width or height, depending the given dimension.
- Throws:
IndexOutOfBoundsException
- if the given index is out of bounds.
-
getMinX
public double getMinX()Returns the minimal coordinate value for dimension 0. The default implementation invokesgetMinimum(0)
. The result is the standardRectangle2D
value (namely x) only if the envelope is not crossing the anti-meridian.- Overrides:
getMinX
in classRectangularShape
- Returns:
- the minimal coordinate value for dimension 0.
-
getMinY
public double getMinY()Returns the minimal coordinate value for dimension 1. The default implementation invokesgetMinimum(1)
. The result is the standardRectangle2D
value (namely y) only if the envelope is not crossing the anti-meridian.- Overrides:
getMinY
in classRectangularShape
- Returns:
- the minimal coordinate value for dimension 1.
-
getMaxX
public double getMaxX()Returns the maximal coordinate value for dimension 0. The default implementation invokesgetMinimum(0)
. The result is the standardRectangle2D
value (namely x + width) only if the envelope is not crossing the anti-meridian.- Overrides:
getMaxX
in classRectangularShape
- Returns:
- the maximal coordinate value for dimension 0.
-
getMaxY
public double getMaxY()Returns the maximal coordinate value for dimension 1. The default implementation invokesgetMinimum(1)
. The result is the standardRectangle2D
value (namely y + height) only if the envelope is not crossing the anti-meridian.- Overrides:
getMaxY
in classRectangularShape
- Returns:
- the maximal coordinate value for dimension 1.
-
getCenterX
public double getCenterX()Returns the median coordinate value for dimension 0. The default implementation invokesgetMedian(0)
. The result is the standardRectangle2D
value (namely x + width/2) only if the envelope is not crossing the anti-meridian.- Overrides:
getCenterX
in classRectangularShape
- Returns:
- the median coordinate value for dimension 0.
-
getCenterY
public double getCenterY()Returns the median coordinate value for dimension 1. The default implementation invokesgetMedian(1)
. The result is the standardRectangle2D
value (namely y + height/2) only if the envelope is not crossing the anti-meridian.- Overrides:
getCenterY
in classRectangularShape
- Returns:
- the median coordinate value for dimension 1.
-
getWidth
public double getWidth()Returns the span for dimension 0. The default implementation invokesgetSpan(0)
. The result is the standardRectangle2D
value (namely width) only if the envelope is not crossing the anti-meridian.- Overrides:
getWidth
in classRectangle2D.Double
- Returns:
- the span for dimension 0.
-
getHeight
public double getHeight()Returns the span for dimension 1. The default implementation invokesgetSpan(1)
. The result is the standardRectangle2D
value (namely height) only if the envelope is not crossing the anti-meridian.- Overrides:
getHeight
in classRectangle2D.Double
- Returns:
- the span for dimension 1.
-
isEmpty
public boolean isEmpty()Determines whether the envelope is empty. A negative Rectangle2D.Double.width or (@linkplain #height} is considered as a non-empty area if the corresponding axis has the wraparound range meaning.Note that if the Rectangle2D.Double.width or Rectangle2D.Double.height value is
NaN
, then the envelope is considered empty. This is different than the defaultRectangle2D.Double.isEmpty()
implementation, which doesn't check forNaN
values.- Specified by:
isEmpty
in interfaceEmptiable
- Overrides:
isEmpty
in classRectangle2D.Double
- Returns:
true
if this envelope is empty.
-
toRectangles
Returns this envelope as non-empty Java2D rectangle objects. This method returns an array of length 0, 1, 2 or 4 depending on whether the envelope crosses the anti-meridian or the limit of any other axis having wraparound range meaning. More specifically:- If this envelope is empty, then this method returns an empty array.
- If this envelope does not have any wraparound behavior, then this method returns a copy
of this envelope as an instance of
Rectangle2D.Double
in an array of length 1. - If this envelope crosses the anti-meridian (a.k.a. date line) then this method represents this envelope as two separated rectangles.
- While uncommon, the envelope could theoretically crosses the limit of other axis having wraparound range meaning. If wraparound occur along the two axes, then this method represents this envelope as four separated rectangles.
API note: The return type is theRectangle2D.Double
implementation class rather than theRectangle2D
abstract class because theEnvelope2D
class hierarchy already exposes this implementation choice.- Returns:
- a representation of this envelope as an array of non-empty Java2D rectangles.
The array never contains
this
. - Since:
- 0.4
- See Also:
-
contains
public boolean contains(double px, double py) Tests if a specified coordinate is inside the boundary of this envelope. If it least one of the given coordinate value isNaN
, then this method returnsfalse
.Crossing the anti-meridian of a Geographic CRS
This method supports anti-meridian in the same way thanAbstractEnvelope.contains(DirectPosition)
.- Specified by:
contains
in interfaceShape
- Overrides:
contains
in classRectangle2D
- Parameters:
px
- the first coordinate value of the point to text.py
- the second coordinate value of the point to text.- Returns:
true
if the specified coordinate is inside the boundary of this envelope;false
otherwise.
-
contains
Returnstrue
if this envelope completely encloses the specified rectangle. If this envelope or the given rectangle have at least oneNaN
value, then this method returnsfalse
.Crossing the anti-meridian of a Geographic CRS
This method supports anti-meridian in the same way thanAbstractEnvelope.contains(Envelope)
.- Specified by:
contains
in interfaceShape
- Overrides:
contains
in classRectangularShape
- Parameters:
rect
- the rectangle to test for inclusion.- Returns:
true
if this envelope completely encloses the specified rectangle.
-
contains
public boolean contains(double rx, double ry, double rw, double rh) Returnstrue
if this envelope completely encloses the specified rectangle. If this envelope or the given rectangle have at least oneNaN
value, then this method returnsfalse
.Crossing the anti-meridian of a Geographic CRS
This method supports anti-meridian in the same way thanAbstractEnvelope.contains(Envelope)
.- Specified by:
contains
in interfaceShape
- Overrides:
contains
in classRectangle2D
- Parameters:
rx
- the x coordinate of the lower corner of the rectangle to test for inclusion.ry
- the y coordinate of the lower corner of the rectangle to test for inclusion.rw
- the width of the rectangle to test for inclusion. May be negative if the rectangle spans the anti-meridian.rh
- the height of the rectangle to test for inclusion. May be negative.- Returns:
true
if this envelope completely encloses the specified one.
-
intersects
Returnstrue
if this envelope intersects the specified envelope. If this envelope or the given rectangle have at least oneNaN
value, then this method returnsfalse
.Crossing the anti-meridian of a Geographic CRS
This method supports anti-meridian in the same way thanAbstractEnvelope.intersects(Envelope)
.- Specified by:
intersects
in interfaceShape
- Overrides:
intersects
in classRectangularShape
- Parameters:
rect
- the rectangle to test for intersection.- Returns:
true
if this envelope intersects the specified rectangle.
-
intersects
public boolean intersects(double rx, double ry, double rw, double rh) Returnstrue
if this envelope intersects the specified envelope. If this envelope or the given rectangle have at least oneNaN
value, then this method returnsfalse
.Crossing the anti-meridian of a Geographic CRS
This method supports anti-meridian in the same way thanAbstractEnvelope.intersects(Envelope)
.- Specified by:
intersects
in interfaceShape
- Overrides:
intersects
in classRectangle2D
- Parameters:
rx
- the x coordinate of the lower corner of the rectangle to test for intersection.ry
- the y coordinate of the lower corner of the rectangle to test for intersection.rw
- the width of the rectangle to test for inclusion. May be negative if the rectangle spans the anti-meridian.rh
- the height of the rectangle to test for inclusion. May be negative.- Returns:
true
if this envelope intersects the specified rectangle.
-
createIntersection
Returns the intersection of this envelope with the specified rectangle. If this envelope or the given rectangle have at least oneNaN
values, then this method returns an empty envelope.Crossing the anti-meridian of a Geographic CRS
This method supports anti-meridian in the same way thanGeneralEnvelope.intersect(Envelope)
.- Overrides:
createIntersection
in classRectangle2D.Double
- Parameters:
rect
- the rectangle to be intersected with this envelope.- Returns:
- the intersection of the given rectangle with this envelope.
-
createUnion
Returns the union of this envelope with the specified rectangle. The default implementation clones this envelope, then delegates toadd(Rectangle2D)
.- Overrides:
createUnion
in classRectangle2D.Double
- Parameters:
rect
- the rectangle to add to this envelope.- Returns:
- the union of the given rectangle with this envelope.
-
add
Adds another rectangle to this rectangle. The resulting rectangle is the union of the twoRectangle
objects.Crossing the anti-meridian of a Geographic CRS
This method supports anti-meridian in the same way thanGeneralEnvelope.add(Envelope)
, except if the result is a rectangle expanding to infinities. In that later case, the field values are set toNaN
because infinite values are a problematic inRectangle2D
objects.- Overrides:
add
in classRectangle2D
- Parameters:
rect
- the rectangle to add to this envelope.
-
add
public void add(double px, double py) Adds a point to this rectangle. The resulting rectangle is the smallest rectangle that contains both the original rectangle and the specified point.After adding a point, a call to
contains(double, double)
with the added point as an argument will returntrue
, except if one of the point coordinates wasDouble.NaN
in which case the corresponding coordinate has been ignored.Crossing the anti-meridian of a Geographic CRS
This method supports anti-meridian in the same way thanGeneralEnvelope.add(DirectPosition)
.- Overrides:
add
in classRectangle2D
- Parameters:
px
- the first coordinate of the point to add.py
- the second coordinate of the point to add.
-
equals
Compares the specified object with this envelope for equality. If the given object is not an instance ofEnvelope2D
, then the two objects are compared as plain rectangles, i.e. the coordinate reference system of this envelope is ignored.Note on
This class does not override thehashCode()
Rectangle2D.hashCode()
method for consistency with theRectangle2D.equals(Object)
method, which compare arbitraryRectangle2D
implementations.- Overrides:
equals
in classRectangle2D
- Parameters:
object
- the object to compare with this envelope.- Returns:
true
if the given object is equal to this envelope.
-
boundsEquals
Returnstrue
ifthis
envelope bounds is equal tothat
envelope bounds in two specified dimensions. The coordinate reference system is not compared, since it doesn't need to have the same number of dimensions.- Parameters:
that
- the envelope to compare to.xDim
- the dimension ofthat
envelope to compare to the x dimension ofthis
envelope.yDim
- the dimension ofthat
envelope to compare to the y dimension ofthis
envelope.eps
- a small tolerance number for floating point number comparisons. This value will be scaled according this envelope width and height.- Returns:
true
if the envelope bounds are the same (up to the specified tolerance level) in the specified dimensions, orfalse
otherwise.
-
clone
Returns a clone of this envelope.- Overrides:
clone
in classRectangularShape
- Returns:
- a clone of this envelope.
-
toString
Formats this envelope as a "BOX
" element. The output is of the form "BOX(
lower corner,
upper corner)
". Example:BOX(-90 -180, 90 180)
- Overrides:
toString
in classRectangle2D.Double
- See Also:
-