- All Implemented Interfaces:
Serializable
,Cloneable
,Emptiable
,Envelope
Envelope
(a minimum bounding box or rectangle) of arbitrary dimension.
Regardless of dimension, an Envelope
can be represented without ambiguity
as two direct positions (coordinate tuples).
To encode an Envelope
, it is sufficient to encode these two points.
Envelope
uses an arbitrary Coordinate Reference System, which does not need to be geographic.
This is different than the GeographicBoundingBox
class provided in the metadata package, which can be used
as a kind of envelope restricted to a Geographic CRS having Greenwich prime meridian.
Envelope
is said "General" because it uses
coordinates of an arbitrary number of dimensions. This is in contrast with
Envelope2D
, which can use only two-dimensional coordinates.
A GeneralEnvelope
can be created in various ways:
- From a given number of dimension, with all coordinates initialized to 0.
- From two coordinate tuples.
- From a another envelope (copy constructor).
- From a geographic bounding box.
- From a character sequence
representing a
BBOX
or a Well Known Text (WKT) format.
Crossing the anti-meridian of a Geographic CRS
The Web Coverage Service (WCS) specification authorizes (with special treatment) cases where upper < lower at least in the longitude case. They are envelopes crossing the anti-meridian, like the red box below (the green box is the usual case). The default implementation of methods listed in the right column can handle such cases.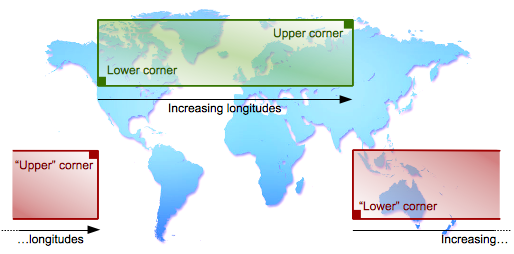
AbstractEnvelope.getMinimum(int)
AbstractEnvelope.getMaximum(int)
AbstractEnvelope.getMedian(int)
AbstractEnvelope.getSpan(int)
AbstractEnvelope.isEmpty()
toSimpleEnvelopes()
contains(DirectPosition)
contains(Envelope)
intersects(Envelope)
intersect(Envelope)
add(Envelope)
add(DirectPosition)
Envelope validation
If and only if this envelope is associated to a non-null CRS, then constructors and setter methods in this class perform the following checks:- The number of CRS dimensions must be equal to
this.Envelope.getDimension()
. - For each dimension i,
getLower(i) > getUpper(i)
is allowed only if the coordinate system axis range meaning isWRAPAROUND
.
add(…)
, intersect(…)
, contains(…)
and similar methods.
This in contrast with the lower > upper
case, which cause the above-cited methods to behave in
an unexpected way if the axis does not have wraparound range meaning.- Since:
- 0.3
- See Also:
-
Constructor Summary
ConstructorsConstructorDescriptionGeneralEnvelope
(double[] lowerCorner, double[] upperCorner) Constructs an envelope defined by two corners given as sequences of coordinate values.GeneralEnvelope
(int dimension) Constructs an empty envelope of the specified dimension.Constructs a new envelope initialized to the values parsed from the given string inBOX
or Well Known Text (WKT) format.GeneralEnvelope
(DirectPosition lowerCorner, DirectPosition upperCorner) Constructs an envelope defined by two corners given as direct positions.GeneralEnvelope
(Envelope envelope) Constructs a new envelope with the same data than the specified envelope.Constructs a new envelope with the same data than the specified geographic bounding box.Constructs an empty envelope with the specified coordinate reference system. -
Method Summary
Modifier and TypeMethodDescriptionvoid
add
(DirectPosition position) Adds a point to this envelope.void
Adds an envelope object to this envelope.static GeneralEnvelope
castOrCopy
(Envelope envelope) Returns the given envelope as aGeneralEnvelope
instance.clone()
Returns a deep copy of this envelope.boolean
Returnstrue
if the specified object is an envelope of the same class with equals coordinates and CRS.Returns the envelope coordinate reference system, ornull
if unknown.int
Returns the length of coordinate sequence (the number of entries) in this envelope.double
getLower
(int dimension) Returns the limit in the direction of decreasing coordinate values in the specified dimension.double
getMaximum
(int dimension) Returns the maximal coordinate value for the specified dimension.double
getMedian
(int dimension) Returns the median coordinate along the specified dimension.double
getMinimum
(int dimension) Returns the minimal coordinate value for the specified dimension.double
getSpan
(int dimension) Returns the envelope span (typically width or height) along the specified dimension.double
getUpper
(int dimension) Returns the limit in the direction of increasing coordinate values in the specified dimension.int
Returns a hash value for this envelope.Returns a view over the two horizontal dimensions of this envelope.void
Sets this envelope to the intersection of this envelope with the specified one.boolean
Returnsfalse
if at least one coordinate value is not NaN.boolean
isEmpty()
Determines whether or not this envelope is empty.boolean
Ensures that the envelope is contained inside the coordinate system domain.void
Sets the coordinate reference system in which the coordinate are given.void
setEnvelope
(double... corners) Sets the envelope to the specified values, which must be the lower corner coordinates followed by upper corner coordinates.void
setEnvelope
(Envelope envelope) Sets this envelope to the same coordinate values than the specified envelope.void
setRange
(int dimension, double lower, double upper) Sets the envelope range along the specified dimension.boolean
setTimeRange
(Instant startTime, Instant endTime) If this envelope has a temporal component, sets its temporal dimension to the given range.void
Sets the lower corner to negative infinity and the upper corner to positive infinity.void
Sets all coordinate values to NaN.boolean
simplify()
Ensures that lower ≤ upper for every dimensions.subEnvelope
(int beginIndex, int endIndex) Returns a view over this envelope that encompass only some dimensions.Formats this envelope as a "BOX
" element.void
translate
(double... vector) Translates the envelope by the given vector.boolean
wraparound
(WraparoundMethod method) If this envelope is crossing the limit of a wraparound axis, modifies coordinates by application of the specified strategy.Methods inherited from class AbstractEnvelope
contains, contains, contains, equals, formatTo, getLowerCorner, getMedian, getSpan, getTimeRange, getUpperCorner, intersects, intersects, isFinite, toSimpleEnvelopes
Methods inherited from class FormattableObject
print, toString, toWKT
-
Constructor Details
-
GeneralEnvelope
public GeneralEnvelope(DirectPosition lowerCorner, DirectPosition upperCorner) throws MismatchedDimensionException, MismatchedReferenceSystemException Constructs an envelope defined by two corners given as direct positions. If at least one corner is associated to a CRS, then the new envelope will also be associated to that CRS.- Parameters:
lowerCorner
- the limits in the direction of decreasing coordinate values for each dimension.upperCorner
- the limits in the direction of increasing coordinate values for each dimension.- Throws:
MismatchedDimensionException
- if the two positions do not have the same dimension.MismatchedReferenceSystemException
- if the CRS of the two position are not equal.
-
GeneralEnvelope
public GeneralEnvelope(double[] lowerCorner, double[] upperCorner) throws MismatchedDimensionException Constructs an envelope defined by two corners given as sequences of coordinate values. The Coordinate Reference System is initiallynull
.- Parameters:
lowerCorner
- the limits in the direction of decreasing coordinate values for each dimension.upperCorner
- the limits in the direction of increasing coordinate values for each dimension.- Throws:
MismatchedDimensionException
- if the two sequences do not have the same length.
-
GeneralEnvelope
public GeneralEnvelope(int dimension) Constructs an empty envelope of the specified dimension. All coordinates are initialized to 0 and the coordinate reference system is undefined.- Parameters:
dimension
- the envelope dimension.
-
GeneralEnvelope
Constructs an empty envelope with the specified coordinate reference system. All coordinate values are initialized to 0.- Parameters:
crs
- the coordinate reference system.
-
GeneralEnvelope
Constructs a new envelope with the same data than the specified envelope.- Parameters:
envelope
- the envelope to copy.- See Also:
-
GeneralEnvelope
Constructs a new envelope with the same data than the specified geographic bounding box. The coordinate reference system is set to the default geographic CRS. Axis order is (longitude, latitude).- Parameters:
box
- the bounding box to copy.
-
GeneralEnvelope
Constructs a new envelope initialized to the values parsed from the given string inBOX
or Well Known Text (WKT) format. The given string is typically aBOX
element like below:BOX(-180 -90, 180 90)
POLYGON
. Actually this constructor ignores the geometry type and just applies the following simple rules:- Character sequences complying to the rules of Java identifiers are skipped.
- Coordinates are separated by a coma (
,
) character. - The coordinates in a coordinate tuple are separated by a space.
- Coordinate numbers are assumed formatted in US locale.
- The coordinate having the highest dimension determines the dimension of this envelope.
LINESTRING
have the same dimension. However, this constructor ensures that the parenthesis are balanced, in order to catch some malformed WKT.Example
The following texts can be parsed by this constructor in addition of the usualBOX
element. This constructor creates the bounding box of those geometries:POINT(6 10)
MULTIPOLYGON(((1 1, 5 1, 1 5, 1 1),(2 2, 3 2, 3 3, 2 2)))
GEOMETRYCOLLECTION(POINT(4 6),LINESTRING(3 8,7 10))
- Parameters:
wkt
- theBOX
,POLYGON
or other kind of element to parse.- Throws:
IllegalArgumentException
- if the given string cannot be parsed.- See Also:
-
-
Method Details
-
castOrCopy
Returns the given envelope as aGeneralEnvelope
instance. If the given envelope is already an instance ofGeneralEnvelope
, then it is returned unchanged. Otherwise the coordinate values and the CRS of the given envelope are copied in a newGeneralEnvelope
.- Parameters:
envelope
- the envelope to cast, ornull
.- Returns:
- the values of the given envelope as a
GeneralEnvelope
instance. - See Also:
-
setCoordinateReferenceSystem
public void setCoordinateReferenceSystem(CoordinateReferenceSystem crs) throws MismatchedDimensionException Sets the coordinate reference system in which the coordinate are given. This method does not reproject the envelope, and does not check if the envelope is contained in the new domain of validity.If the envelope coordinates need to be transformed to the new CRS, consider using
Envelopes.transform(Envelope, CoordinateReferenceSystem)
instead.- Parameters:
crs
- the new coordinate reference system, ornull
.- Throws:
MismatchedDimensionException
- if the specified CRS doesn't have the expected number of dimensions.IllegalStateException
- if a range of coordinate values in this envelope is compatible with the given CRS. See Envelope validation in class javadoc for more details.
-
setRange
Sets the envelope range along the specified dimension.- Parameters:
dimension
- the dimension to set.lower
- the limit in the direction of decreasing coordinate values.upper
- the limit in the direction of increasing coordinate values.- Throws:
IndexOutOfBoundsException
- if the given index is out of bounds.IllegalArgumentException
- iflower > upper
and the axis range meaning at the given dimension is not "wraparound". See Envelope validation in class javadoc for more details.
-
setEnvelope
public void setEnvelope(double... corners) Sets the envelope to the specified values, which must be the lower corner coordinates followed by upper corner coordinates. The number of arguments provided shall be twice this envelope dimension, and minimum shall not be greater than maximum.Example: (xmin, ymin, zmin, xmax, ymax, zmax)- Parameters:
corners
- coordinates of the new lower corner followed by the new upper corner.
-
setEnvelope
Sets this envelope to the same coordinate values than the specified envelope. If the given envelope has a non-null Coordinate Reference System (CRS), then the CRS of this envelope will be set to the CRS of the given envelope.- Parameters:
envelope
- the envelope to copy coordinates from.- Throws:
MismatchedDimensionException
- if the specified envelope does not have the expected number of dimensions.
-
setToInfinite
public void setToInfinite()Sets the lower corner to negative infinity and the upper corner to positive infinity. The coordinate reference system (if any) stay unchanged. -
setToNaN
public void setToNaN()Sets all coordinate values to NaN. The coordinate reference system (if any) stay unchanged.- See Also:
-
setTimeRange
If this envelope has a temporal component, sets its temporal dimension to the given range. Otherwise this method does nothing. This convenience method converts the given instants to floating point values usingDefaultTemporalCRS
, then delegates tosetRange(int, double, double)
.Null value means no time limit. More specifically null
startTime
is mapped to −∞ and nullendTime
is mapped to +∞. This rule makes easy to create is before or is after temporal filters, which can be combined with other envelopes using intersection for logical AND, or union for logical OR operations.- Parameters:
startTime
- the lower temporal value, ornull
if unbounded.endTime
- the upper temporal value, ornull
if unbounded.- Returns:
- whether the temporal component has been set, or
false
if no temporal dimension has been found in this envelope. - Since:
- 1.0
- See Also:
-
translate
public void translate(double... vector) Translates the envelope by the given vector. For every dimension i, the lower and upper values are increased byvector[i]
.This method does not check if the translation result is inside the coordinate system domain (e.g. [-180 … +180]° of longitude). Callers can normalize the envelope when desired by call to the
normalize()
method.- Parameters:
vector
- the translation vector. The length of this array shall be equal to this envelope dimension.- Since:
- 0.5
-
add
Adds a point to this envelope. The resulting envelope is the smallest envelope that contains both the original envelope and the specified point.After adding a point, a call to
contains(DirectPosition)
with the added point as an argument will returntrue
, except if one of the point coordinates wasDouble.NaN
in which case the corresponding coordinate has been ignored.Preconditions
This method assumes that the specified point uses a CRS equivalent to this envelope CRS. For performance reasons, it will no be verified unless Java assertions are enabled.Crossing the anti-meridian of a Geographic CRS
This method supports envelopes crossing the anti-meridian. In such cases it is possible to move both envelope borders in order to encompass the given point, as illustrated below (the new point is represented by the+
symbol):─────┐ + ┌───── ─────┘ └─────
The default implementation moves only the border which is closest to the given point.- Parameters:
position
- the point to add.- Throws:
MismatchedDimensionException
- if the given point does not have the expected number of dimensions.AssertionError
- if assertions are enabled and the envelopes have mismatched CRS.
-
add
Adds an envelope object to this envelope. The resulting envelope is the union of the twoEnvelope
objects.Preconditions
This method assumes that the specified envelope uses a CRS equivalent to this envelope CRS. For performance reasons, it will no be verified unless Java assertions are enabled.Crossing the anti-meridian of a Geographic CRS
This method supports envelopes crossing the anti-meridian. If one or both envelopes cross the anti-meridian, then the result of theadd
operation may be an envelope expanding to infinities. In such case, the coordinate range will be either [−∞…∞] or [0…−0] depending on whatever the original range crosses the anti-meridian or not.Handling of NaN values
Double.NaN
values may be present in any dimension, in the lower coordinate, upper coordinate or both. The behavior of this method in such case depends where theNaN
values appear and whether an envelope spans the anti-meridian:- If this envelope or the given envelope spans anti-meridian in the dimension containing
NaN
coordinates, then this method does not changes the coordinates in that dimension. The rational for such conservative approach is because union computation depends on whether the other envelope spans anti-meridian too, which is unknown because at least one envelope bounds isNaN
. Since anti-meridian crossing has been detected in an envelope, there is suspicion about whether the other envelope could cross anti-meridian too. - Otherwise since the envelope containing real values does not cross anti-meridian in that dimension,
this method assumes that the envelope containing
NaN
values does not cross anti-meridian neither. This assumption is not guaranteed to be true, but cover common cases. With this assumption in mind:- All
NaN
coordinates in the given envelope are ignored, i.e. this method does not replace finite coordinates in this envelope byNaN
values from the given envelope. Note that if only the lower or upper bound isNaN
, the other bound will still be used for computing union with the assumption described in above paragraph. - All
NaN
coordinates in this envelope are left unchanged, i.e. the union will still contain all theNaN
values that this envelope had beforeadd(Envelope)
invocation. Note that if only the lower or upper bound isNaN
, the other bound will still be used for computing union with the assumption described in above paragraph.
- All
- Parameters:
envelope
- theEnvelope
to add to this envelope.- Throws:
MismatchedDimensionException
- if the given envelope does not have the expected number of dimensions.AssertionError
- if assertions are enabled and the envelopes have mismatched CRS.- See Also:
- If this envelope or the given envelope spans anti-meridian in the dimension containing
-
intersect
Sets this envelope to the intersection of this envelope with the specified one.Preconditions
This method assumes that the specified envelope uses a CRS equivalent to this envelope CRS. For performance reasons, it will no be verified unless Java assertions are enabled.Crossing the anti-meridian of a Geographic CRS
This method supports envelopes crossing the anti-meridian.Handling of NaN values
Double.NaN
values may be present in any dimension, in the lower coordinate, upper coordinate or both. The behavior of this method in such case depends where theNaN
values appear and whether an envelope spans the anti-meridian:- If this envelope or the given envelope spans anti-meridian in the dimension containing
NaN
coordinates, then this method does not changes the coordinates in that dimension. The rational for such conservative approach is because intersection computation depends on whether the other envelope spans anti-meridian too, which is unknown because at least one envelope bounds isNaN
. Since anti-meridian crossing has been detected in an envelope, there is suspicion about whether the other envelope could cross anti-meridian too. - Otherwise since the envelope containing real values does not cross anti-meridian in that dimension,
this method assumes that the envelope containing
NaN
values does not cross anti-meridian neither. This assumption is not guaranteed to be true, but cover common cases. With this assumption in mind:- All
NaN
coordinates in the given envelope are ignored, i.e. this method does not replace finite coordinates in this envelope byNaN
values from the given envelope. Note that if only the lower or upper bound isNaN
, the other bound will still be used for computing intersection with the assumption described in above paragraph. - All
NaN
coordinates in this envelope are left unchanged, i.e. the intersection will still contain all theNaN
values that this envelope had beforeintersect(Envelope)
invocation. Note that if only the lower or upper bound isNaN
, the other bound will still be used for computing intersection with the assumption described in above paragraph.
- All
Double.NaN
coordinates may appear as a result of intersection, even if such values were not present in any source envelopes, if the two envelopes do not intersect in some dimensions.- Parameters:
envelope
- theEnvelope
to intersect to this envelope.- Throws:
MismatchedDimensionException
- if the given envelope does not have the expected number of dimensions.AssertionError
- if assertions are enabled and the envelopes have mismatched CRS.- See Also:
- If this envelope or the given envelope spans anti-meridian in the dimension containing
-
normalize
public boolean normalize()Ensures that the envelope is contained inside the coordinate system domain. For each dimension, this method compares the coordinate values against the limits of the coordinate system axis for that dimension. If some coordinates are out of range, then there is a choice depending on the axis range meaning:- If
RangeMeaning.EXACT
(typically latitudes coordinates), then values greater than the axis maximal value are replaced by the axis maximum, and values smaller than the axis minimal value are replaced by the axis minimum. - If
RangeMeaning.WRAPAROUND
(typically longitudes coordinates), then a multiple of the axis range (e.g. 360° for longitudes) is added or subtracted. Example:- the [190 … 200]° longitude range is converted to [-170 … -160]°,
- the [170 … 200]° longitude range is converted to [+170 … -160]°.
Crossing the anti-meridian of a Geographic CRS
If the envelope is crossing the anti-meridian, then some lower coordinate values may become greater than their upper counterpart as a result of this method call. If such effect is undesirable, then this method may be combined withsimplify()
as below:if (envelope.normalize()) { envelope.simplify(); }
Choosing the range of longitude values
Geographic CRS typically have longitude values in the [-180 … +180]° range, but the [0 … 360]° range is also occasionally used. Callers need to ensure that this envelope CRS is associated to axes having the desired minimum and maximum value.Usage
This method is sometimes useful before to compute the union or intersection of envelopes, in order to ensure that both envelopes are defined in the same domain. This method may also be invoked before to project an envelope, since some projections produceDouble.NaN
numbers when given an coordinate value out of bounds.- Returns:
true
if this envelope has been modified as a result of this method call, orfalse
if no change has been done.- See Also:
- If
-
simplify
Ensures that lower ≤ upper for every dimensions. If a upper coordinate value is less than a lower coordinate value, then there is a choice:- If the axis has
RangeMeaning.WRAPAROUND
, then:- the lower coordinate value is set to the axis minimum value, and
- the upper coordinate value is set to the axis maximum value.
- Otherwise an
IllegalStateException
is thrown.
- Returns:
true
if this envelope has been modified as a result of this method call, orfalse
if no change has been done.- Throws:
IllegalStateException
- if a upper coordinate value is less than a lower coordinate value on an axis which does not have theWRAPAROUND
range meaning.- See Also:
- If the axis has
-
wraparound
If this envelope is crossing the limit of a wraparound axis, modifies coordinates by application of the specified strategy. This applies typically to longitude values crossing the anti-meridian, but other kinds of wraparound axes may also exist. Possible values are listed below.Legal argument values Value Action WraparoundMethod.NONE
:Do nothing and return false
.WraparoundMethod.NORMALIZE
:Delegate to normalize()
.WraparoundMethod.EXPAND
:Equivalent to simplify()
.WraparoundMethod.CONTIGUOUS
:See enumeration javadoc. WraparoundMethod.CONTIGUOUS_LOWER
:See enumeration javadoc. WraparoundMethod.CONTIGUOUS_UPPER
:See enumeration javadoc. WraparoundMethod.SPLIT
:Throw IllegalArgumentException
.- Parameters:
method
- the strategy to use for representing a region crossing the anti-meridian or other wraparound limit.- Returns:
true
if this envelope has been modified as a result of this method call, orfalse
if no change has been done.- Throws:
IllegalStateException
- if a upper coordinate value is less than a lower coordinate value on an axis which does not have theWRAPAROUND
range meaning.- Since:
- 1.1
-
horizontal
Returns a view over the two horizontal dimensions of this envelope. The horizontal dimensions are inferred from the CRS. If this method cannot infer the horizontal dimensions, then anIllegalStateException
is thrown.The returned envelope is a view: changes in the returned envelope are reflected in this envelope, and conversely. The returned envelope will have its CRS defined.
- Returns:
- a view over the horizontal components of this envelope. May be
this
. - Throws:
IllegalStateException
- if this method cannot infer the horizontal components of this envelope.- Since:
- 1.1
- See Also:
-
subEnvelope
Returns a view over this envelope that encompass only some dimensions. The returned object is "live": changes applied on the original envelope is reflected in the sub-envelope view, and conversely.This method is useful for querying and updating only some dimensions. For example, in order to expand only the horizontal component of a four dimensional (x,y,z,t) envelope, one can use:
envelope.subEnvelope(0, 2).add(myPosition2D);
GeneralEnvelope copy = envelope.subEnvelope(0, 2).clone();
null
CRS. This method does not compute a sub-CRS because it may not be needed, or the sub-CRS may be already known by the caller.- Parameters:
beginIndex
- the index of the first valid coordinate value of the corners.endIndex
- the index after the last valid coordinate value of the corners.- Returns:
- the sub-envelope of dimension
endIndex - beginIndex
. - Throws:
IndexOutOfBoundsException
- if an index is out of bounds.- See Also:
-
clone
Returns a deep copy of this envelope. -
getDimension
public int getDimension()Returns the length of coordinate sequence (the number of entries) in this envelope. This information is available even when the coordinate reference system is unknown.- Specified by:
getDimension
in interfaceEnvelope
- Returns:
- the dimensionality of this envelope.
-
getCoordinateReferenceSystem
Returns the envelope coordinate reference system, ornull
if unknown. If non-null, it shall be the same as lower corner and upper corner CRS.- Specified by:
getCoordinateReferenceSystem
in interfaceEnvelope
- Returns:
- the envelope CRS, or
null
if unknown.
-
getLower
Returns the limit in the direction of decreasing coordinate values in the specified dimension. This is usually the algebraic minimum, except if this envelope spans the anti-meridian.- Specified by:
getLower
in classAbstractEnvelope
- Parameters:
dimension
- the dimension for which to obtain the coordinate value.- Returns:
- the starting coordinate value at the given dimension.
- Throws:
IndexOutOfBoundsException
- if the given index is negative or is equal or greater than the envelope dimension.- See Also:
-
getUpper
Returns the limit in the direction of increasing coordinate values in the specified dimension. This is usually the algebraic maximum, except if this envelope spans the anti-meridian.- Specified by:
getUpper
in classAbstractEnvelope
- Parameters:
dimension
- the dimension for which to obtain the coordinate value.- Returns:
- the starting coordinate value at the given dimension.
- Throws:
IndexOutOfBoundsException
- if the given index is negative or is equal or greater than the envelope dimension.- See Also:
-
getMinimum
Returns the minimal coordinate value for the specified dimension. In the typical case of non-empty envelopes not crossing the anti-meridian, this method returns theAbstractEnvelope.getLower(int)
value verbatim. In the case of envelope crossing the anti-meridian, this method returns the axis minimum value. If the range in the given dimension is invalid, then this method returnsNaN
.- Specified by:
getMinimum
in interfaceEnvelope
- Overrides:
getMinimum
in classAbstractEnvelope
- Parameters:
dimension
- the dimension for which to obtain the coordinate value.- Returns:
- the minimal coordinate value at the given dimension.
- Throws:
IndexOutOfBoundsException
- if the given index is negative or is equal or greater than the envelope dimension.
-
getMaximum
Returns the maximal coordinate value for the specified dimension. In the typical case of non-empty envelopes not crossing the anti-meridian, this method returns theAbstractEnvelope.getUpper(int)
value verbatim. In the case of envelope crossing the anti-meridian, this method returns the axis maximum value. If the range in the given dimension is invalid, then this method returnsNaN
.- Specified by:
getMaximum
in interfaceEnvelope
- Overrides:
getMaximum
in classAbstractEnvelope
- Parameters:
dimension
- the dimension for which to obtain the coordinate value.- Returns:
- the maximal coordinate value at the given dimension.
- Throws:
IndexOutOfBoundsException
- if the given index is negative or is equal or greater than the envelope dimension.
-
getMedian
Returns the median coordinate along the specified dimension. In most cases, the result is equal (minus rounding error) to:median = (getUpper(dimension) + getLower(dimension)) / 2;
Crossing the anti-meridian of a Geographic CRS
If upper < lower and the range meaning for the requested dimension is wraparound, then the median calculated above is actually in the middle of the space outside the envelope. In such cases, this method shifts the median value by half of the periodicity (180° in the longitude case) in order to switch from outer space to inner space. If the axis range meaning is notWRAPAROUND
, then this method returnsNaN
.- Specified by:
getMedian
in interfaceEnvelope
- Overrides:
getMedian
in classAbstractEnvelope
- Parameters:
dimension
- the dimension for which to obtain the coordinate value.- Returns:
- the median coordinate at the given dimension, or
Double.NaN
. - Throws:
IndexOutOfBoundsException
- if the given index is negative or is equal or greater than the envelope dimension.- See Also:
-
getSpan
Returns the envelope span (typically width or height) along the specified dimension. In most cases, the result is equal (minus rounding error) to:span = getUpper(dimension) - getLower(dimension);
Crossing the anti-meridian of a Geographic CRS
If upper < lower and the range meaning for the requested dimension is wraparound, then the span calculated above is negative. In such cases, this method adds the periodicity (typically 360° of longitude) to the span. If the result is a positive number, it is returned. Otherwise this method returnsNaN
.- Specified by:
getSpan
in interfaceEnvelope
- Overrides:
getSpan
in classAbstractEnvelope
- Parameters:
dimension
- the dimension for which to obtain the span.- Returns:
- the span (typically width or height) at the given dimension, or
Double.NaN
. - Throws:
IndexOutOfBoundsException
- if the given index is negative or is equal or greater than the envelope dimension.
-
isEmpty
public boolean isEmpty()Determines whether or not this envelope is empty. An envelope is empty if it has zero dimension, or if the span of at least one axis is negative, 0 orNaN
.Note: Strictly speaking, there is an ambiguity if a span isIfNaN
or if the envelope contains both 0 and infinite spans (since 0⋅∞ =NaN
). In such cases, this method arbitrarily ignores the infinite values and returnstrue
.isEmpty()
returnsfalse
, thenAbstractEnvelope.isAllNaN()
is guaranteed to also returnfalse
. However, the converse is not always true.- Specified by:
isEmpty
in interfaceEmptiable
- Overrides:
isEmpty
in classAbstractEnvelope
- Returns:
true
if this envelope is empty.- See Also:
-
isAllNaN
public boolean isAllNaN()Returnsfalse
if at least one coordinate value is not NaN. ThisisAllNaN()
check is different than theAbstractEnvelope.isEmpty()
check since it returnsfalse
for a partially initialized envelope, whileisEmpty()
returnsfalse
only after all dimensions have been initialized. More specifically, the following rules apply:- If
isAllNaN() == true
, thenisEmpty() == true
- If
isEmpty() == false
, thenisAllNaN() == false
- The converse of the above-cited rules are not always true.
- Overrides:
isAllNaN
in classAbstractEnvelope
- Returns:
true
if this envelope has NaN values.- See Also:
- If
-
hashCode
public int hashCode()Returns a hash value for this envelope.- Overrides:
hashCode
in classAbstractEnvelope
-
equals
Returnstrue
if the specified object is an envelope of the same class with equals coordinates and CRS.Implementation note
This implementation requires that the providedobject
argument is of the same class than this envelope. We do not relax this rule since not every implementations in the SIS code base follow the same contract.- Overrides:
equals
in classAbstractEnvelope
- Parameters:
object
- the object to compare with this envelope.- Returns:
true
if the given object is equal to this envelope.
-
toString
Formats this envelope as a "BOX
" element. The output is of the form "BOX
nD(
lower corner,
upper corner)
" where n is the number of dimensions. The number of dimension is written only if different than 2. Examples:BOX(-90 -180, 90 180)
BOX3D(-90 -180 0, 90 180 1)
Double.toString(double)
(i.e. without fixed number of fraction digits). The string returned by this method can be parsed by theGeneralEnvelope
constructor.Note on standards
TheBOX
element is not part of the standard Well Known Text (WKT) format. However, it is understood by many software libraries, for example GDAL and PostGIS.- Overrides:
toString
in classAbstractEnvelope
- Returns:
- this envelope as a
BOX
orBOX3D
(most typical dimensions) element.
-